How to Use the @Data Annotation of Project Lombok
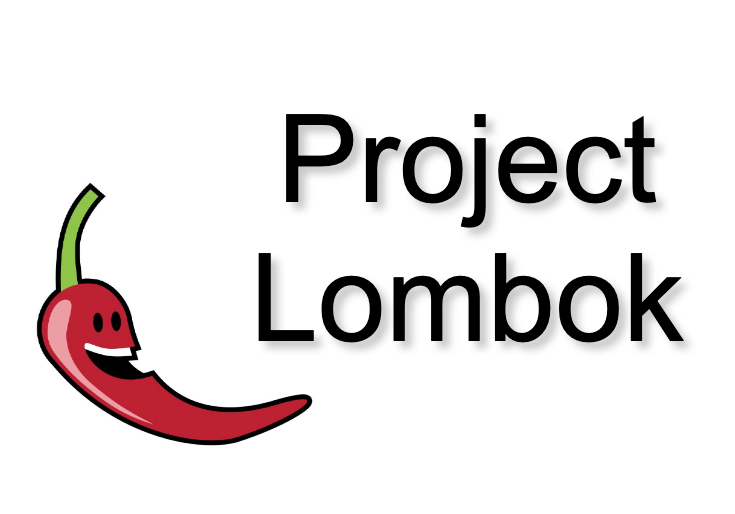
This article explains how to make use of the @Data annotation of Project Lombok.
This post will focus on how to make use of the @Data
annotation of Project Lombok. The Lombok Project is a java library that helps a developer generate boilerplate code. By simply adding the Lombok library to your IDE and build path the Lombok library will auto-generate the Java bytecode, as per the annotations, into the class files.
The @Data
annotation is logically equivalent to adding the @ToString
, @EqualsAndHashCode
, @Getter
, @Setter
and @RequiredArgsConstructor
to your class.
Article Series
This article forms part of a multi-part series on how to use the Project Lombok to generate boilerplate code in java projects.
- How to Use the @AllArgsConstructor Annotation of Project Lombok
- How to Use the @Builder Annotation of Project Lombok
- How to Use the @CleanUp Annotation of Project Lombok
- How to Use the @Data Annotation of Project Lombok
- How to Use the @EqualsAndHashCode Annotation of Project Lombok
- How to Use the @Getter and @Setter Annotations of Project Lombok
Define Maven Dependencies
The following dependencies should be included in the pom.xml
file.
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.8</version>
<scope>provided</scope>
</dependency>
Example: @Data
The @Data annotation generated boilerplate code associated with beans and Java POJOs. These include getters and setters for all the fields, an equals and hashcode method, a toString method , and a constructor to initialize the object.
Original Class
@Data
public class DataExample01 {
private long id;
private String firstName;
private String lastName;
private String gender;
private Date birthDate;
private String contactEmail;
private boolean isFirstYear;
}
To truly appreciate the magic of the Lombok library, you should compile the DataExample01
class by making use of maven to build your project. As part of the compile process, Lombok will generate the boilerplate code depending on the type of annotation you used. To see the boilerplate code, you should decompile DataExample01.class
file. An easy way to do this is to make use of the following URL:
Java Decompiler: http://www.javadecompilers.com
Decompiled Class
public class DataExample01
{
private long id;
private String firstName;
private String lastName;
private String gender;
private Date birthDate;
private String contactEmail;
private boolean isFirstYear;
public long getId() {
return this.id;
}
public String getFirstName() {
return this.firstName;
}
public String getLastName() {
return this.lastName;
}
public String getGender() {
return this.gender;
}
public Date getBirthDate() {
return this.birthDate;
}
public String getContactEmail() {
return this.contactEmail;
}
public boolean isFirstYear() {
return this.isFirstYear;
}
public void setId(final long id) {
this.id = id;
}
public void setFirstName(final String firstName) {
this.firstName = firstName;
}
public void setLastName(final String lastName) {
this.lastName = lastName;
}
public void setGender(final String gender) {
this.gender = gender;
}
public void setBirthDate(final Date birthDate) {
this.birthDate = birthDate;
}
public void setContactEmail(final String contactEmail) {
this.contactEmail = contactEmail;
}
public void setFirstYear(final boolean isFirstYear) {
this.isFirstYear = isFirstYear;
}
@Override
public boolean equals(final Object o) {
if (o == this) {
return true;
}
if (!(o instanceof DataExample01)) {
return false;
}
final DataExample01 other = (DataExample01)o;
if (!other.canEqual(this)) {
return false;
}
if (this.getId() != other.getId()) {
return false;
}
final Object this$firstName = this.getFirstName();
final Object other$firstName = other.getFirstName();
Label_0079: {
if (this$firstName == null) {
if (other$firstName == null) {
break Label_0079;
}
}
else if (this$firstName.equals(other$firstName)) {
break Label_0079;
}
return false;
}
final Object this$lastName = this.getLastName();
final Object other$lastName = other.getLastName();
Label_0116: {
if (this$lastName == null) {
if (other$lastName == null) {
break Label_0116;
}
}
else if (this$lastName.equals(other$lastName)) {
break Label_0116;
}
return false;
}
final Object this$gender = this.getGender();
final Object other$gender = other.getGender();
Label_0153: {
if (this$gender == null) {
if (other$gender == null) {
break Label_0153;
}
}
else if (this$gender.equals(other$gender)) {
break Label_0153;
}
return false;
}
final Object this$birthDate = this.getBirthDate();
final Object other$birthDate = other.getBirthDate();
Label_0190: {
if (this$birthDate == null) {
if (other$birthDate == null) {
break Label_0190;
}
}
else if (this$birthDate.equals(other$birthDate)) {
break Label_0190;
}
return false;
}
final Object this$contactEmail = this.getContactEmail();
final Object other$contactEmail = other.getContactEmail();
if (this$contactEmail == null) {
if (other$contactEmail == null) {
return this.isFirstYear() == other.isFirstYear();
}
}
else if (this$contactEmail.equals(other$contactEmail)) {
return this.isFirstYear() == other.isFirstYear();
}
return false;
}
protected boolean canEqual(final Object other) {
return other instanceof DataExample01;
}
@Override
public int hashCode() {
final int PRIME = 59;
int result = 1;
final long $id = this.getId();
result = result * 59 + (int)($id >>> 32 ^ $id);
final Object $firstName = this.getFirstName();
result = result * 59 + (($firstName == null) ? 43 : $firstName.hashCode());
final Object $lastName = this.getLastName();
result = result * 59 + (($lastName == null) ? 43 : $lastName.hashCode());
final Object $gender = this.getGender();
result = result * 59 + (($gender == null) ? 43 : $gender.hashCode());
final Object $birthDate = this.getBirthDate();
result = result * 59 + (($birthDate == null) ? 43 : $birthDate.hashCode());
final Object $contactEmail = this.getContactEmail();
result = result * 59 + (($contactEmail == null) ? 43 : $contactEmail.hashCode());
result = result * 59 + (this.isFirstYear() ? 79 : 97);
return result;
}
@Override
public String toString() {
return "DataExample01(id=" + this.getId() + ", firstName=" + this.getFirstName() + ", lastName=" + this.getLastName() + ", gender=" + this.getGender() + ", birthDate=" + this.getBirthDate() + ", contactEmail=" + this.getContactEmail() + ", isFirstYear=" + this.isFirstYear() + ")";
}
}
Summary
Congratulations !!! You have successfully used the @Data
annotation of Project Lombok. Please look out for more examples on how to make use of Project Lombok to simplify your Java coding experience. Follow me on any of the different social media platforms and feel free to leave comments.